本文概述
在本主题中,我们将了解如何在Bash脚本中使用if-else语句来完成我们的自动化任务。
Bash if-else语句用于在语句的顺序执行流程中执行条件任务。有时,如果条件为真,我们想处理一组特定的语句,如果条件为假,则要处理另一组语句。为了执行此类操作,我们可以应用if-else机制。我们可以通过“ if语句”应用条件。
if-else语法
Bash Shell脚本中if-else语句的语法可以定义如下:
if [ condition ];
then
<if block commands>
else
<else block commands>
fi
要记住的要点
- 我们可以使用一组使用条件运算符连接的一个或多个条件。
- 其他块命令包括一组在条件为假时执行的动作。
- 条件表达式后的分号(;)是必须的。
查看以下示例,演示在Bash脚本中使用if-else语句:
例子1
下面的示例包含两个不同的场景,其中在第一个if-else语句中条件为true,在第二个if-else语句中条件为false。
#!/bin/bash
#when the condition is true
if [ 10 -gt 3 ];
then
echo "10 is greater than 3."
else
echo "10 is not greater than 3."
fi
#when the condition is false
if [ 3 -gt 10 ];
then
echo "3 is greater than 10."
else
echo "3 is not greater than 10."
fi
输出量
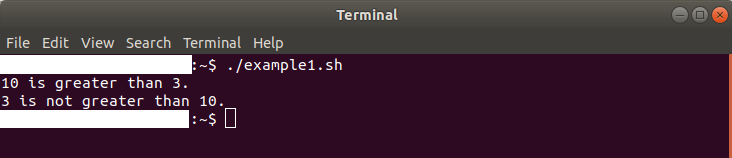
在第一个if-else表达式中,条件(10 -gt 3)为true,因此执行if块中的语句。而在另一个if-else表达式中,条件(3 -gt 10)为false,因此执行else块中的语句。
例子2
在此示例中,我们说明了如何在Bash中的if-else语句中使用多个条件。我们使用bash逻辑运算符来加入多个条件。
#!/bin/bash
# When condition is true
# TRUE && FALSE || FALSE || TRUE
if [[ 10 -gt 9 && 10 == 9 || 2 -lt 1 || 25 -gt 20 ]];
then
echo "Given condition is true."
else
echo "Given condition is false."
fi
# When condition is false
#TRUE && FALSE || FALSE || TRUE
if [[ 10 -gt 9 && 10 == 8 || 3 -gt 4 || 8 -gt 8 ]];
then
echo "Given condition is true."
else
echo "Given condition is not true."
fi
输出量
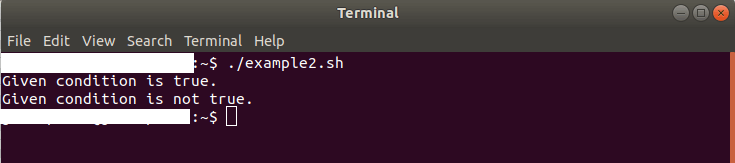
如果在一行中没有其他语句,请立即执行Bash
我们可以在一行中编写完整的“ if-else语句”以及命令。你需要遵循给定的规则,才能在一行中使用if-else语句:
- 在if和else块的语句末尾使用分号(;)。
- 使用空格作为分隔符来附加所有语句。
下面给出一个示例,说明如何在单行中使用if-else语句:
例
#!/bin/bash
read -p "Enter a value:" value
if [ $value -gt 9 ]; then echo "The value you typed is greater than 9."; else echo "The value you typed is not greater than 9."; fi
输出量
当我们输入值25时,输出将如下所示:
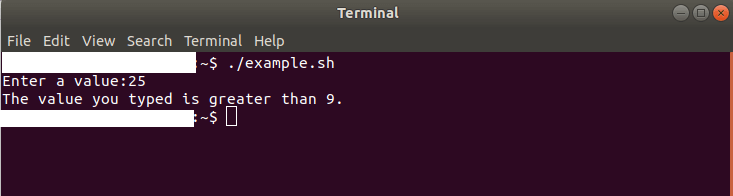
bash if-else嵌套
就像嵌套的if语句一样,if-else语句也可以在另一个if-else语句中使用。在Bash脚本中将其称为嵌套if-else。
以下是一个示例,解释了如何在Bash中使用嵌套的if-else语句:
例
#!/bin/bash
read -p "Enter a value:" value
if [ $value -gt 9 ];
then
if [ $value -lt 11 ];
then
echo "$value>9, $value<11"
else
echo "The value you typed is greater than 9."
fi
else echo "The value you typed is not greater than 9."
fi
输出量
如果我们输入10作为值,那么输出将如下所示:
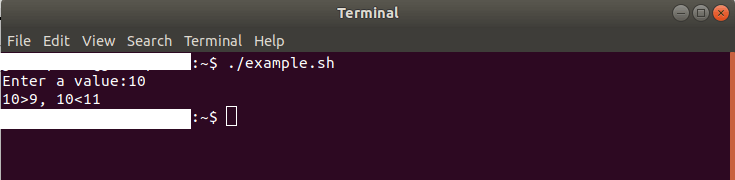
结论
在本主题中,我们通过示例了解了Bash if-else语句的语法和用法。
评论前必须登录!
注册