上一章TypeScript教程请查看:TypeScript集合set用法
与其他编程语言一样,Typescript允许我们在类级使用访问修饰符。它为类成员提供直接访问控制。这些类成员是函数和属性。我们可以在它自己的类内部、类之外的任何地方、或者在它的子类或派生类中使用类成员。
访问修饰符增加了类成员的安全性,并防止它们被非法使用。我们还可以使用它来控制类的数据成员的可见性。如果类不需要设置任何访问修饰符,TypeScript会自动设置所有类成员的公共访问修饰符。
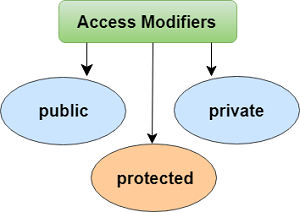
TypeScript访问修饰符有三种类型。这些都是:
- 公共的public
- 私有的private
- 保护的protected
理解所有TypeScript访问修饰符
让我们了解一下给定表的访问修饰符。
访问修饰符 | 类内部 | 子类 | 可通过类实例从外部访问 |
Public | Yes | Yes | Yes |
Protected | Yes | Yes | No |
Private | Yes | No | No |
公共的Public
默认情况下,在TypeScript中,类的所有成员(属性和方法)都是公共的。因此,不需要在成员前面加上这个关键字。我们可以在任何地方不受任何限制地访问这个数据成员。
例子
class Student {
public studCode: number;
studName: string;
}
let stud = new Student();
stud.studCode = 101;
stud.studName = "Oreja";
console.log(stud.studCode+ " "+stud.studName);
在上面的例子中,studCode是公共的,studName声明时没有修饰符,因此TypeScript默认将它们视为公共的。由于数据成员是公共的,所以可以使用类的对象在类外部访问它们。
私有的private
私有访问修饰符不能在其包含的类之外访问。它确保类成员仅对其所在的类可见。
例子
class Student {
public studCode: number;
private studName: string;
constructor(code: number, name: string){
this.studCode = code;
this.studName = name;
}
public display() {
return (`unique code: ${this.studCode}, my name: ${this.studName}.`);
}
}
let student: Student = new Student(1, "Oreja");
console.log(student.display());
在上面的例子中,studCode是私有的,studName的声明没有修饰符,所以TypeScript默认将它作为公共的。如果我们在类外部访问私有成员,它将给出一个编译错误。
受保护的protected
受保护的访问修饰符只能在类及其子类中访问,我们不能从它所在的类的外部访问它。
例子
class Student {
public studCode: number;
protected studName: string;
constructor(code: number, name: string){
this.studCode = code;
this.studName = name;
}
}
class Person extends Student {
private department: string;
constructor(code: number, name: string, department: string) {
super(code, name);
this.department = department;
}
public getElevatorPitch() {
return (`unique code: ${this.studCode}, my name: ${this.studName} and I am in ${this.department} Branch.`);
}
}
let joeRoot: Person = new Person(1, "Oreja", "CS");
console.log(joeRoot.getElevatorPitch());
在上面的例子中,我们不能使用学生类之外的名称。我们仍然可以在Person类的实例方法中使用它,因为Person类派生自Student类。
只读修饰符readonly
- 我们可以使用readonly修饰符使类、类型或接口的属性为只读。
- 此修饰符需要在其声明时或在构造函数中初始化。
- 我们也可以从类的外部访问只读成员,但是它的值不能改变。
例子
class Company {
readonly country: string = "UK";
readonly name: string;
constructor(contName: string) {
this.name = contName;
}
showDetails() {
console.log(this.name + " : " + this.country);
}
}
let comp = new Company("srcmini");
comp.showDetails();
comp.name = "TCS"; //Error, 只能在内部初始化
评论前必须登录!
注册